Binary trees play an essential role in computer science, facilitating efficient data storage and manipulation. Understanding the fundamentals of binary tree structures and how to manipulate them is crucial for developing optimized algorithms and data structures. One specific operation, swapping the left and right children of each node, is commonly used in algorithms like tree mirroring and data structure optimization. In this article, we will delve into how binary trees work and focus on swapping their left and right children, with a focus on coding and performance considerations.
What is a Binary Tree?
A binary tree is a hierarchical data structure in which each node has at most two children, referred to as the left and right child. The tree’s top node is called the root node, and each node is connected by edges. The primary utility of binary trees is their hierarchical arrangement, which makes them ideal for use in various applications such as searching, sorting, and hierarchical data representation.
Types of Binary Trees
There are several types of binary trees based on specific characteristics, such as:
- Full Binary Tree: Each node has either 0 or 2 children.
- Perfect Binary Tree: All interior nodes have two children, and all leaf nodes are at the same level.
- Complete Binary Tree: All levels are completely filled except possibly the last level, which is filled from left to right.
- Balanced Binary Tree: The height difference between the left and right subtrees is minimal, usually by one level.
Importance of the Root Node
The root node is the cornerstone of a binary tree. It serves as the starting point for traversing the entire tree, guiding access to all other nodes. Swapping the left and right children of nodes can have a profound impact on the tree’s overall structure and behavior, especially during traversal operations like in-order, pre-order, and post-order traversals.
Read Also: TypeError: ‘str’ object does not support item assignment

Why Swap the Left and Right Children of Each Node?
Swapping the left and right children of each node is a common operation performed to create a mirror image of the tree. This technique is particularly useful in several algorithmic applications:
- Symmetry Detection: Checking if two binary trees are mirror images of each other.
- Tree Reconstruction: Constructing new trees from existing structures in reverse order.
- Data Transformation: Changing the orientation of data representation for more efficient processing.
By swapping the children, we alter the tree’s traversal order and potentially its storage layout, which can influence search and sort algorithms.
How to Swap the Left and Right Children in a Binary Tree
Swapping the left and right children of every node in a binary tree can be achieved through recursion or iterative methods. The recursion-based approach is the most intuitive, but it is essential to consider performance optimizations, especially for larger trees.
Recursive Approach to Swap Children
The recursive approach leverages the divide-and-conquer principle to traverse the entire binary tree and swap the children at each node.
Here’s the step-by-step breakdown of the algorithm:
- Base Case: If the node is
null
, return the node (i.e., there is no operation to be done). - Recursive Case: Swap the left and right children of the current node.
- Traverse Recursively: Call the function recursively on the left child and then on the right child (or vice versa depending on the desired traversal order).
class Node:
def __init__(self, value):
self.value = value
self.left = None
self.right = None
def swap_children(root):
if root is None:
return None
# Swap the left and right children
root.left, root.right = root.right, root.left
# Recursively call the swap on the children
swap_children(root.left)
swap_children(root.right)
return root
This function starts from the root node and recursively traverses the tree. At each step, it swaps the left and right children until it reaches the leaf nodes.
Iterative Approach to Swap Children
An alternative to the recursive method is to use an iterative approach, which may be preferable for deeper trees to avoid stack overflow issues associated with recursion.
Here’s an iterative version of the algorithm using a queue for a level-order traversal:
from collections import deque
def iterative_swap_children(root):
if root is None:
return None
queue = deque([root])
while queue:
node = queue.popleft()
# Swap the children
node.left, node.right = node.right, node.left
# Enqueue children for future swapping
if node.left:
queue.append(node.left)
if node.right:
queue.append(node.right)
return root
The iterative approach uses a queue to traverse the tree level by level. As it visits each node, it swaps the children, ensuring that all levels are processed correctly.
Read Also: TypeError: ‘type’ object is not subscriptable
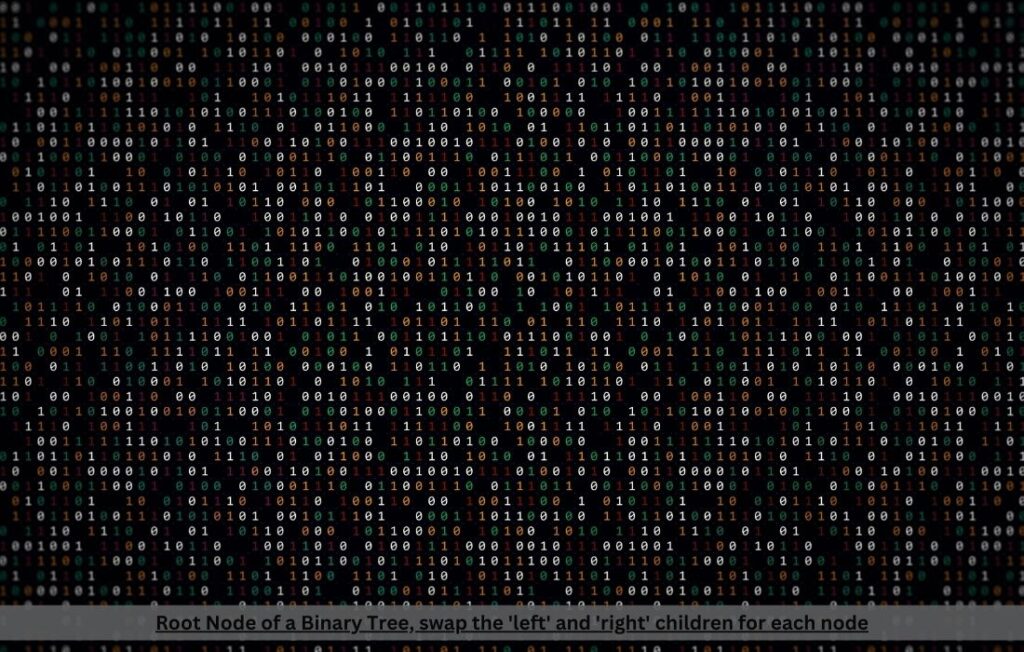
Applications of Swapping Left and Right Children
Swapping the left and right children of a binary tree is not just a theoretical exercise—it has real-world applications across a variety of domains. Some of the common applications include:
- Image Processing: Trees are used in digital image processing, where mirrored versions of image data can be generated by swapping nodes in binary trees representing image pixels.
- Symmetric Tree Validation: In validation algorithms, the symmetry of a tree can be determined by comparing it with its mirror image created by swapping children.
- Data Compression: Binary trees are widely used in data compression algorithms, and child-swapping operations may be utilized during tree restructuring phases.
- Artificial Intelligence (AI) Algorithms: Binary trees are essential in AI search algorithms, where swapping nodes may be part of exploring different tree configurations.
Performance Considerations
Swapping the left and right children of every node in a binary tree may seem like a straightforward operation, but performance can be a concern for trees with a large number of nodes. The complexity of this operation is O(n), where n
is the number of nodes in the tree. Both the recursive and iterative approaches have this complexity, but recursion can lead to stack overflow in cases where the tree depth is significant.
Optimizing Recursive Depth
If the binary tree has a deep structure, especially in cases where the tree is unbalanced, recursion might hit the stack limit. To prevent this, consider tail recursion optimizations or use an iterative approach when working with very large trees. Another consideration is memory usage, as the recursive approach holds multiple stack frames simultaneously.
Balanced Trees for Efficient Swapping
Binary trees that are more balanced will benefit from more efficient traversal during the child-swapping operation. It is recommended to keep binary trees balanced when possible to optimize the swapping process, whether it’s done recursively or iteratively.
Read Also: typeerror: not all arguments converted during string formatting
Conclusion
Swapping the left and right children in a binary tree is an essential operation with far-reaching implications in algorithm design and real-world applications. Whether used for creating a mirror image of a tree, validating tree symmetry, or optimizing data structures, understanding how to perform this operation efficiently is a critical skill for developers and data scientists.