The TypeError: 'type' object is not subscriptable
is a common Python error that developers often encounter when working with dictionaries, lists, or type hints. This error occurs when you mistakenly use square brackets []
on a type object like list
, dict
, or type
, rather than on an instance of that type. Understanding the causes and solutions for TypeError: 'type' object is not subscriptable
can help you avoid it and keep your code running smoothly.
What Causes TypeError: ‘type’ Object is Not Subscriptable?
The TypeError 'type' object is not subscriptable
typically arises when you try to access or manipulate a type object as if it were subscriptable. Here are common scenarios where this error occurs:
- Using a Type Instead of an Instance: One of the most frequent causes of
TypeError:'type' object is not subscriptable
is attempting to use a type object likelist
ordict
as if it were an instance. For example:
dict = {} # Intending to create a dictionary instance.
value = dict['key'] # Trying to access a key-value pair.
This code triggers TypeError:'type' object is not subscriptable
because dict
is incorrectly assigned, leading to confusion between the dict
type and a dictionary instance. The correct approach is shown below:
my_dict = {} # Correctly defining a dictionary instance.
value = my_dict['key'] # Accessing a key from the dictionary instance.
Incorrect Usage of Type Hints: Another common source of TypeError:'type' object is not subscriptable
is using type hints incorrectly in Python versions earlier than 3.9. For instance:
dict = {}
value = dict['key'] # This will raise an error because 'dict' is reserved for the type.
This code causes TypeError: 'type' object is not subscriptable
because list[int]
is not allowed in Python versions before 3.9. To avoid this error, use the typing
module:
from typing import List
my_list: List[int] = [1, 2, 3] # Correct type hint usage to avoid TypeError: 'type' object is not subscriptable.
- Confusing Type Annotations with Objects: Misunderstanding type annotations is another frequent reason for encountering
TypeError: 'type' object is not subscriptable
. When using annotations, always remember that they should describe the type of data, not be treated as objects.
Read Also: The Techno Tricks: Transform Your World
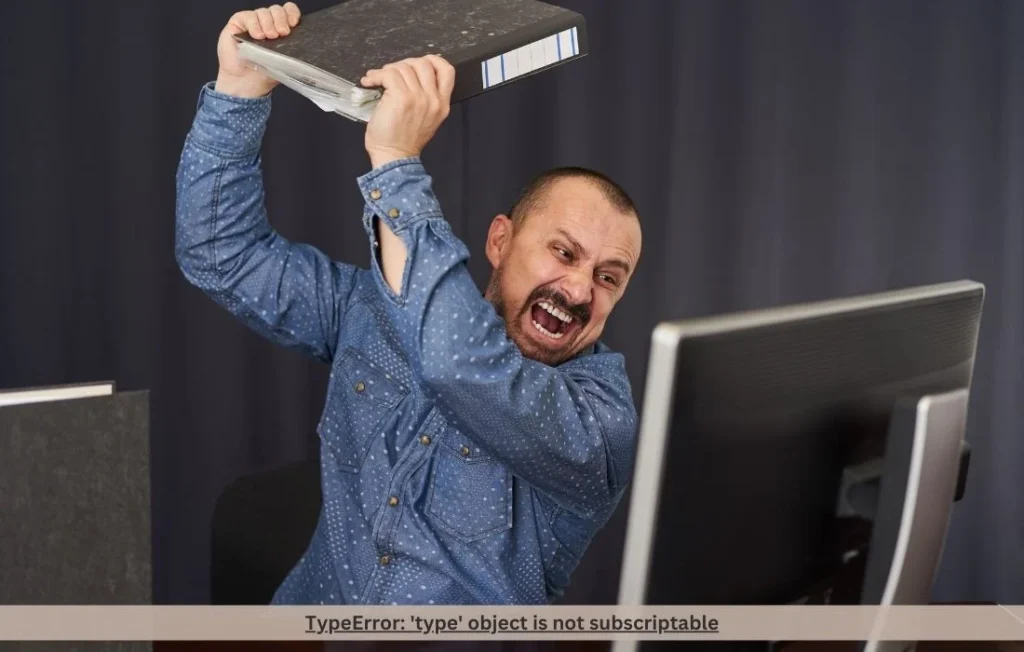
Solution for TypeError: ‘type’ Object is Not Subscriptable
To resolve TypeError: 'type' object is not subscriptable
, follow these steps:
- Check Variable Names: Ensure you are not using type names like
list
,dict
, ortype
as variable names. Misusing these names is a common reason behindTypeError: 'type' object is not subscriptable
. - Use Correct Type Hints: Especially in Python versions below 3.9, always import the correct types from the
typing
module to avoid'type'
. - Avoid Subscribing to Type Objects: Ensure you are accessing instances and not type objects themselves. This will help you steer clear of
type' object is not subscriptable Error
. - Correct Code Example:
dict = {}
value = dict['key'] # This will raise an error because 'dict' is reserved for the type.
Conclusion
Encountering TypeError: 'type' object is not subscriptable
is a common occurrence in Python programming, especially when working with type hints and data structures. This error usually happens when developers confuse type objects with instances. By carefully managing your variables, using type hints correctly, and ensuring you are accessing instances rather than types, you can avoid TypeError: 'type' object is not subscriptable
.
Understanding the underlying causes of 'type' object is not subscriptable
helps prevent frustrating debugging sessions and keeps your code efficient and readable. Paying attention to variable names, especially avoiding conflicts with Python’s built-in types, and applying correct type hinting practices can make your code more robust. Furthermore, familiarizing yourself with Python’s type system and knowing when to use types versus instances will significantly reduce errors like 'type' object is not subscriptable
. As you become more adept at recognizing these pitfalls, your coding skills will grow, allowing you to write cleaner and more reliable Python code that is free from errors like 'type' object is not subscriptable
.